CAMEL 教程:为大规模语言模型探索构建通信代理
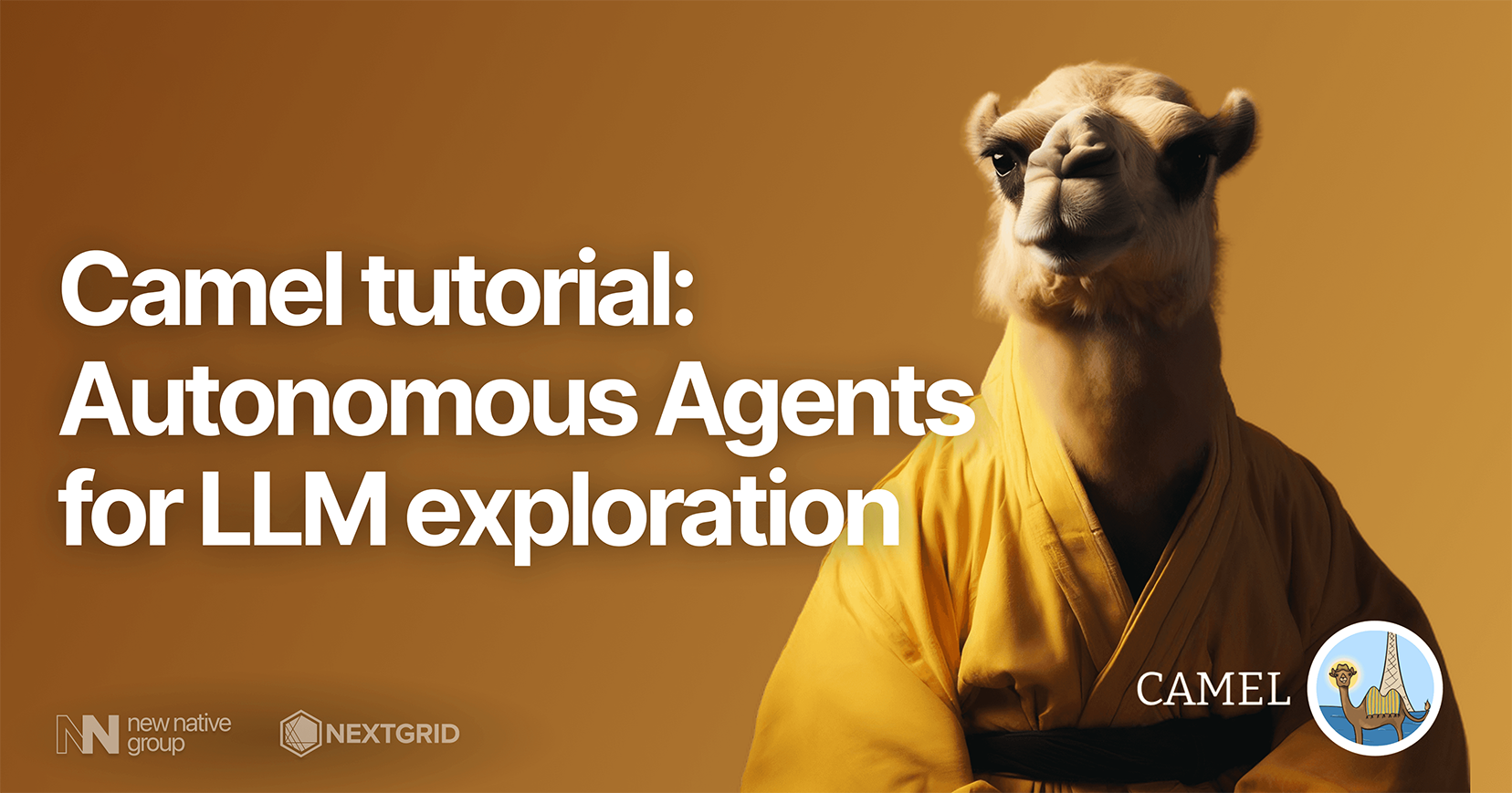
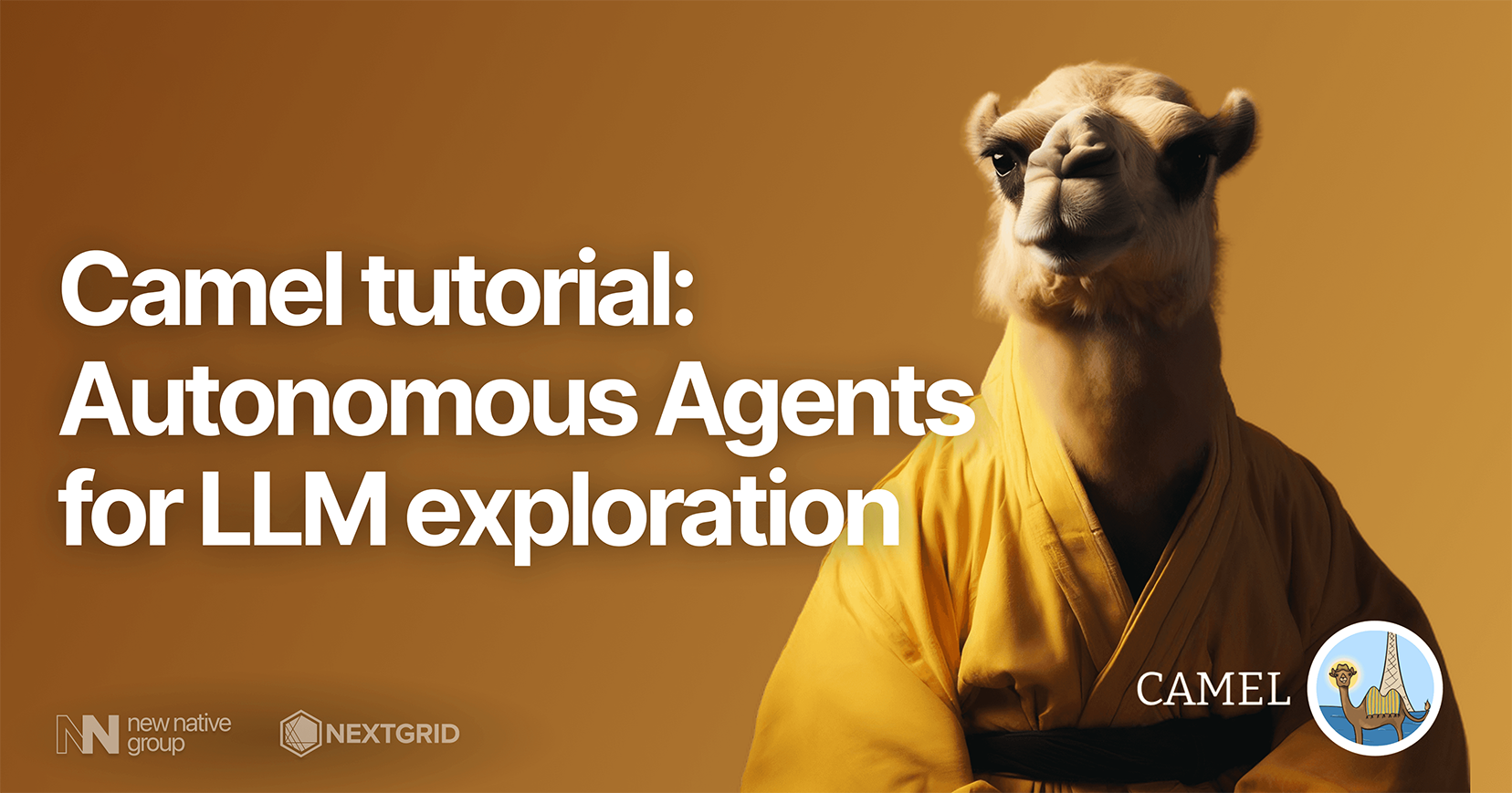
在本教程中,我们将介绍安装、一般信息和使用 CAMEL 的示例,CAMEL 是一种通信代理之间自主合作的框架。CAMEL 是一种新颖的交流代理框架,它利用初始提示来引导聊天代理完成任务,同时保持与人类意图的一致性。它旨在生成用于研究聊天代理的行为和功能的会话数据,为调查会话语言模型提供宝贵的资源。
安装
要安装 CAMEL,请执行以下步骤:
克隆 GitHub 存储库:
git clone https://github.com/lightaime/camel.git
将目录更改为项目目录:
cd camel
从源代码安装 CAMEL:
pre-commit installpip install -e .
深入示例:角色扮演
这个例子演示了如何使用 CAMEL 在两个代理之间进行角色扮演来解决指定的任务。您可以自定义角色、任务和其他参数,以创建不同的场景来研究多智能体系统的协作行为和能力。
第一步:导入必要的模块
我们需要为这个例子导入必要的模块。此外,我们需要添加我们的 OpenAI API 密钥。
import osimport openaiopenai.api_key = "sk-..."
接下来,我们将定义一个小的辅助函数来打印对话的结果。
import timefrom colorama import Foredef print_text_animated(text): for char in text: print(char, end="", flush=True) time.sleep(0.02)
第 2 步:定义角色
现在我们可以定义两个代理的角色。在此示例中,我们将使用计算机程序员和游戏玩家。总体任务是使用 pygame 设计自定义游戏,pygame 是一种流行的用于游戏开发的 Python 库。
from camel.agents import RolePlaying task_prompt = "Design a custom game using pygame"print(Fore.YELLOW + f"Original task prompt:\n{task_prompt}\n")role_play_session = RolePlaying("Computer Programmer", "Gamer", task_prompt)print(Fore.CYAN + f"Specified task prompt:\n{role_play_session.task_prompt}\n")chat_turn_limit, n = 10, 0assistant_msg, _ = role_play_session.init_chat()while n < chat_turn_limit: n += 1 (assistant_msg, _, _), (user_msg, _, _) = role_play_session.step(assistant_msg) print_text_animated(Fore.BLUE + f"AI User:\n\n{user_msg.content}\n\n") print_text_animated(Fore.GREEN + f"AI Assistant:\n\n{assistant_msg.content}\n\n") if "" in user_msg.content: break
Original task prompt:Design a custom game using pygameSpecified task prompt:Design an immersive first-person shooter game using Pygame that includes a variety of challenging levels, power-ups, and unlockable achievements, while also implementing realistic physics simulations and advanced AI enemies to provide players with an unforgettable gaming experience.AI User:Instruction: Install Pygame libraryInput: NoneAI Assistant:Solution: To install Pygame library, open the command prompt or terminal and type "pip install pygame" and press enter. This will download and install the Pygame library on your system. Next request.AI User:Instruction: Create a new Pygame window with a specified width and height.Input: Width and height of the window.AI Assistant:Solution: To create a new Pygame window with a specified width and height, first import the Pygame library and initialize it. Then, create a new window surface using the `pygame.display.set_mode()` function and pass in a tuple containing the width and height of the window as arguments. Finally, set the caption of the window using the `pygame.display.set_caption()` function.Here's an example code snippet:import pygame# Initialize Pygamepygame.init()# Set the width and height of the windowwidth = 800height = 600# Create a new window surfacewindow = pygame.display.set_mode((width, height))# Set the caption of the windowpygame.display.set_caption("My Pygame Window")# Keep the window open until the user closes itwhile True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() sys.exit() pygame.display.update()Next request.AI User:Instruction: Load an image file in Pygame.Input: File path of the image.AI Assistant:Solution: To load an image file in Pygame, use the `pygame.image.load()` function and pass in the file path of the image as an argument. This will return a `Surface` object that you can use to display the image on the screen.Here's an example code snippet:import pygame# Initialize Pygamepygame.init()# Set the width and height of the windowwidth = 800height = 600# Create a new window surfacewindow = pygame.display.set_mode((width, height))# Load an image fileimage_path = "path/to/image.png"image = pygame.image.load(image_path)# Display the image on the screenwindow.blit(image, (0, 0))# Keep the window open until the user closes itwhile True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() sys.exit() pygame.display.update()Next request.AI User:Instruction: Add background music to the game.Input: File path of the music file.AI Assistant:Solution: To add background music to the game, first load the music file using the `pygame.mixer.music.load()` function and pass in the file path of the music as an argument. Then, start playing the music using the `pygame.mixer.music.play()` function. You can also set the volume of the music using the `pygame.mixer.music.set_volume()` function.Here's an example code snippet:import pygame# Initialize Pygamepygame.init()# Set the width and height of the windowwidth = 800height = 600# Create a new window surfacewindow = pygame.display.set_mode((width, height))# Load background musicmusic_path = "path/to/music.mp3"pygame.mixer.music.load(music_path)# Set the volume of the musicpygame.mixer.music.set_volume(0.5)# Start playing the musicpygame.mixer.music.play(-1)# Keep the window open until the user closes itwhile True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() sys.exit() pygame.display.update()Next request.AI User:Instruction: Create a player character sprite that can move around the game window.Input: File path of the player character sprite image.AI Assistant:Solution: To create a player character sprite that can move around the game window, first load the player character sprite image using the `pygame.image.load()` function and pass in the file path of the image as an argument. Then, create a new sprite object using the `pygame.sprite.Sprite()` class and set its image and rect attributes to the loaded image and its initial position on the screen, respectively. Finally, add the sprite to a sprite group and update its position based on user input.Here's an example code snippet:import pygame# Initialize Pygamepygame.init()# Set the width and height of the windowwidth = 800height = 600# Create a new window surfacewindow = pygame.display.set_mode((width, height))# Load the player character sprite imageplayer_image_path = "path/to/player.png"player_image = pygame.image.load(player_image_path)# Create a player sprite objectclass Player(pygame.sprite.Sprite): def __init__(self): super().__init__() self.image = player_image self.rect = self.image.get_rect() self.rect.center = (width // 2, height // 2)# Create a sprite group and add the player sprite to itall_sprites = pygame.sprite.Group()player = Player()all_sprites.add(player)# Keep the window open until the user closes itwhile True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() sys.exit() # Move the player sprite based on user input keys = pygame.key.get_pressed() if keys[pygame.K_LEFT]: player.rect.x -= 5 if keys[pygame.K_RIGHT]: player.rect.x += 5 if keys[pygame.K_UP]: player.rect.y -= 5 if keys[pygame.K_DOWN]: player.rect.y += 5 # Update the sprite group and display the window all_sprites.update() window.fill((255, 255, 255)) all_sprites.draw(window) pygame.display.update()Next request.AI User:Instruction: Create a power-up sprite that the player can collect to increase their score.Input: File path of the power-up sprite image.AI Assistant:Solution: To create a power-up sprite that the player can collect to increase their score, first load the power-up sprite image using the `pygame.image.load()` function and pass in the file path of the image as an argument. Then, create a new sprite object using the `pygame.sprite.Sprite()` class and set its image and rect attributes to the loaded image and its initial position on the screen, respectively. Finally, add the sprite to a sprite group and check for collisions between the player sprite and the power-up sprite to increase the player's score.Here's an example code snippet:import pygame# Initialize Pygamepygame.init()# Set the width and height of the windowwidth = 800height = 600# Create a new window surfacewindow = pygame.display.set_mode((width, height))# Load the player character sprite imageplayer_image_path = "path/to/player.png"player_image = pygame.image.load(player_image_path)# Load the power-up sprite imagepowerup_image_path = "path/to/powerup.png"powerup_image = pygame.image.load(powerup_image_path)# Create a player sprite objectclass Player(pygame.sprite.Sprite): def __init__(self): super().__init__() self.image = player_image self.rect = self.image.get_rect() self.rect.center = (width // 2, height // 2)# Create a power-up sprite objectclass Powerup(pygame.sprite.Sprite): def __init__(self): super().__init__() self.image = powerup_image self.rect = self.image.get_rect() self.rect.center = (400, 300)# Create sprite groups and add the player and power-up sprites to themall_sprites = pygame.sprite.Group()player = Player()powerup = Powerup()all_sprites.add(player, powerup)# Set the initial score to 0score = 0# Keep the window open until the user closes itwhile True: for event in pygame.event.get(): if event.type == pygame.QUIT: pygame.quit() sys.exit() # Move the player sprite based on user input keys = pygame.key.get_pressed() if keys[pygame.K_LEFT]: player.rect.x -= 5 if keys[pygame.K_RIGHT]: player.rect.x += 5 if keys[pygame.K_UP]: player.rect.y -= 5 if keys[pygame.K_DOWN]: player.rect.y += 5 # Check for collisions between the player and power-up sprites if pygame.sprite.collide_rect(player, powerup): powerup.rect.center = (800, 600) # Move the power-up offscreen score += 1 # Update the sprite group and display the window all_sprites.update() window.fill((255, 255, 255)) all_sprites.draw(window) # Display the score on the screen font = pygame.font.Font(None, 36) score_text = font.render("Score: " + str(score), True, (0, 0, 0)) window.blit(score_text, (10, 10)) pygame.display.update()Next request.AI User:Instruction: Create an AI enemy sprite that moves towards the player and damages them on collision.Input: File path of the enemy sprite image.AI Assistant:Solution: To create an AI enemy sprite that moves towards the player and damages them on collision, first load the enemy sprite image using the `pygame.image.load()` function and pass in the file path of the image as an argument. Then, create a new sprite object using the `pygame.sprite.Sprite()` class and set its image and rect attributes to the loaded image and its initial position on the screen, respectively. Finally, add the sprite to a sprite group and update its position based on the player's position and check for collisions between the player sprite and the enemy sprite to decrease the player's health.Here's an example code snippet:import pygame# Initialize Pygamepygame.init()# Set the width and height of the windowwidth = 800height = 600# Create a new window surfacewindow = pygame.display.set_mode((width, height))...
您可以看到代理以合作对话的方式工作来完成任务。您可以在我们的 lablab.ai Github 上找到笔记本以快速入门。
您可以在活动页面上找到即将举行的黑客马拉松和活动。
谢谢你!– AI未来百科 ; 探索AI的边界与未来! 懂您的AI未来站